Best Practices in React Native Development for Cross-Platform Performance
- Samantha Blake
- May 8
- 10 min read
Did you know that studies consistently show users expect mobile applications to load within 2-3 seconds, a delay of even a few hundred milliseconds leading to significant drops in engagement? In the perpetually competitive digital landscape, mobile app performance is not merely a desirable feature; it stands as an imperative requirement. For developers leveraging React Native to build cross-platform performance applications, navigating the inherent complexities to ensure speed and responsiveness on both iOS and Android presents a nuanced challenge. Creating seamless, performant user experiences across diverse devices and operating systems necessitates a meticulous approach. It demands understanding the fundamental architecture of React Native, recognizing common performance bottlenecks, and wielding the right tools and techniques to ameliorate these issues proactively. This guide offers a pragmatic overview of best practices, common pitfalls to circumvent, and strategies that elevate your React Native performance endeavors.
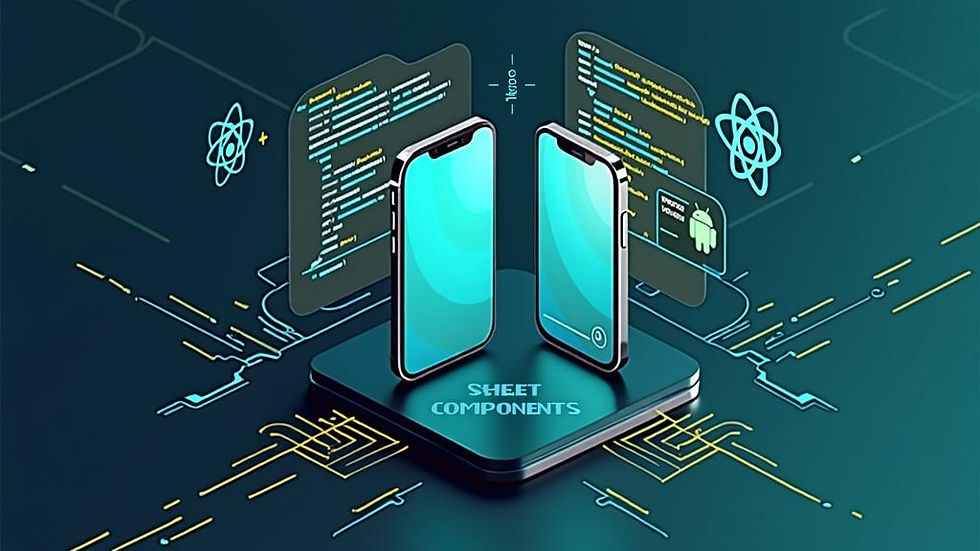
Understanding the Core Challenges
While React Native offers a rapid development cycle and a single codebase for multiple platforms, its architecture introduces specific performance considerations. At its heart lies the bridge – the mechanism facilitating communication between the JavaScript thread and the native UI threads. This bridge, while ingenious, can become a bottleneck if misused or overloaded, leading to sluggish UIs or non-responsive interactions, commonly referred to as "jank." Teams providing Android app development services must be particularly mindful of these architectural intricacies to maintain performance parity across devices.
Bridging the Gap the JS Native Divide
Every interaction, data transfer, or UI update that spans the divide between JavaScript logic and native components incurs overhead due to serialization deserialization. Frequent or large data transfers across this bridge can impede cross-platform performance, creating delays perceivable by the user. Synchronous calls over the bridge are particularly recalcitrant sources of blocking the UI thread.
UI Rendering Bottlenecks
Efficiently rendering and updating the user interface is paramount. In React Native, render performance hinges on how components manage their state props and how effectively re-renders are controlled. Deep component trees or frequent, unnecessary re-renders can tax the JavaScript thread, delaying updates to the native UI. Ensuring optimal rendering cycles is critical for smooth animations and responsive UIs, contributing significantly to perceived mobile app performance.
Memory Leaks Resource Consumption
Poorly managed memory can lead to app crashes, sluggishness, and excessive battery drain. Common culprits include unsubscribed event listeners, retained references to large objects, or inefficient image handling. Identifying and rectifying memory issues is a perpetual task in ensuring robust React Native performance.
Essential Strategies for Peak Performance
Elevating your React Native development practice involves integrating performance considerations throughout the development lifecycle, not merely addressing them post-hoc. A plethora of strategies exists to ameliorate performance issues.
Profiling Your Application Bottlenecks
Before optimizing, you must understand where performance issues reside. Profiling is non-negotiable. Tools like Flipper, the built-in Chrome Debugger (for JS profiling), React Native Debugger, and platform-specific tools like Xcode Instruments (iOS) Android Studio Profiler are invaluable.
Flipper: A ubiquitous debugger platform for mobile apps. It provides network inspection, layout hierarchy, database inspection, Native logs (LogCat console.log), Metro logs, React DevTools, Hermes debugger access, performance monitoring. The Network plugin is vital for identifying slow or excessive API calls. The React DevTools plugin helps inspect component trees state props, revealing unnecessary renders.
Chrome Debugger (legacy) React Native Debugger: Connects to the JavaScript thread, allowing inspection of logs, network requests, performance timings using the Performance tab (though bridge communication isn't fully represented).
Xcode Instruments Android Studio Profiler: These are crucial for profiling native performance aspects, including CPU usage, memory allocation, network activity, and UI rendering specifically on the native threads. Bridging performance issues often manifest here as delays or spikes.
I remember spending days chasing a perceived UI lag, only to profile the app with Flipper and realize the slowdown stemmed entirely from inefficient data processing happening synchronously on the JS thread. The bottleneck wasn't rendering; it was the preceding data manipulation. Profiling provides that kind of undeniable clarity.
Optimizing Component Rendering Lifecycle
Preventing unnecessary re-renders is fundamental. React Native optimization heavily relies on judicious use of component lifecycle methods or their modern equivalents.
Functional Components `useMemo` `useCallback`: Prefer functional components where appropriate. The `useMemo` hook memoizes values to avoid expensive recalculations on every render. The `useCallback` hook memoizes functions themselves, particularly useful when passing callbacks to child components props to prevent the child from re-rendering because a new function instance is created on each parent render.
React.memo: A higher-order component for functional components, akin to `PureComponent` for class components. It shallowly compares props skips re-rendering if props haven't changed. `React.memo` combined with `useCallback` is a powerful combination for preventing prop drilling related re-renders.
Virtualized Lists (`FlatList`, `SectionList` LargeList): For long lists, virtualization is non-negotiable for acceptable React Native performance. These components render only items currently visible plus a few offscreen, recycling views as the user scrolls. Using standard `ScrollView` with many items will lead to severe performance degradation due to excessive memory usage creation of offscreen components. Configure props like `getItemLayout`, `removeClippedSubviews`, `maxToRenderPerBatch`, `updateCellsBatchingPeriod` judiciously based on list item complexity height.
Efficient State Management Practices
How your application manages state can significantly impact performance. While global state libraries like Redux offer structure, inefficient state updates can trigger widespread re-renders.
Granular State Updates: State updates should be as granular as possible, affecting only the components that truly need to re-render. Selectors in Redux (like Reselect) compute derived data efficiently prevent unnecessary re-renders. Newer, simpler state libraries like Zustand Jotai might offer better performance out-of-the-box for simpler use cases due to less boilerplate optimized rendering approaches, but selection depends on project complexity.
Context API Caveats: While convenient for localized state, Context API updates can re-render all consumers even if only a small part of the context value changes. Consider splitting context into smaller, related pieces or using libraries that optimize Context consumption.
Data Fetching Storage Considerations
Slow data fetching, excessive API calls, or inefficient data storage strategies can bottleneck your application.
Data Caching: Implement caching strategies using libraries like React Query RTK Query. These libraries handle caching, revalidation, stale data in a performant manner, reducing loading spinners improving perceived performance.
Offline Capabilities Syncing: For apps requiring offline access, libraries like Realm SQLite offer efficient local data storage. Design syncing mechanisms that minimize network payload optimize data reconciliation, especially for background processes that should not impede UI performance.
Image Optimization: Images are notorious resource hogs. Use optimized image formats (WebP where supported), appropriately sized images (don't load full-resolution images that will only be displayed small), caching libraries like `react-native-fast-image` which leverages native image loading components with enhanced caching options.
Leveraging Native Modules Prudently
For computationally intensive tasks or functionalities requiring direct access to native device features not exposed by React Native's core API, writing native modules in Objective-C/Swift (iOS) Java/Kotlin (Android) is necessary.
Identify Performance Bottlenecks: Only rewrite functionality as a native module when profiling identifies it as a significant bottleneck and it's computationally bound in a way that JS cannot handle efficiently (e.g., complex image processing, heavy data encryption, certain background tasks).
Asynchronous Communication: Always prioritize asynchronous communication when calling native modules from JavaScript to avoid blocking the JS thread. Promises Callbacks are standard patterns. Avoid synchronous bridge calls at all costs.
A particularly enlightening project involved processing large video files. The initial JavaScript amelioration attempts were futile, resulting in minutes of processing time and ANRs (Application Not Responding) on Android. Rewriting the core processing logic as native modules reduced the time to seconds was the singular pragmatic approach.
Code Splitting Bundle Size Management
Larger application bundles lead to longer startup times because the entire JavaScript bundle must be loaded parsed. Keeping the bundle size in check improves the initial loading experience.
Code Splitting: While full code splitting is not as mature as in web applications, strategies like dynamic `import()` (depending on Metro configurations RN version) or routing libraries that support lazy loading components can help load code chunks only when they are needed.
Asset Optimization: Minimize image sizes, use SVG where feasible, cleanup unused assets resources.
Hermes Engine: Using the Hermes JavaScript engine significantly improves startup time memory usage by pre-compiling JavaScript code to bytecode. This is now the default but warrants confirmation verification in your project settings.
Common Pitfalls Avoiding Performance Degradation
Knowing what not to do is as crucial as knowing what to do for peak React Native performance. Certain development patterns are notorious for causing issues.
Excessive Re-renders Deep Component Trees
As mentioned earlier, unchecked re-renders are a ubiquitous issue. Rendering deeply nested component trees or those with extensive data passed via props can quickly overwhelm the rendering pipeline if not carefully managed with `React.memo`, `useMemo`, `useCallback`. A personal observation is that developers often over-memoize out of fear after hitting performance issues; a meticulous approach based on profiling is superior to guess-work.
Blocking the JavaScript Thread
Performing long-running synchronous operations on the JavaScript thread will freeze the UI, making it unresponsive. This includes complex data transformations, heavy computations, or synchronous bridge calls. These should always be moved to background threads or handled via asynchronous operations promises.
Inefficient Image Handling Asset Loading
Failing to use optimized images or specialized image libraries can severely impact performance memory consumption. Loading excessively large images into `Image` components without resizing is a guaranteed way to introduce memory pressure jank.
Over-reliance on the Bridge Synchronous Calls
Making frequent synchronous calls over the bridge ties up both the JavaScript native threads, halting other operations. Any operation requiring significant native work must be asynchronous. This might seem obvious, but it is a frequent slip-up, especially in rapidly developing features.
Tools Techniques for Performance Monitoring Optimization
Effective performance management relies on continuous monitoring iteration. The right tools transform performance tuning from a nebulous task into a targeted effort.
Deep Dive into Profiling Tools
Going beyond basic usage of Flipper or debuggers:
Hermes Debugger: When using Hermes, its debugger is the appropriate tool for stepping through JavaScript code analyzing its execution profile.
React Profiler: Part of React DevTools (accessible via Flipper React Native Debugger), the Profiler tab visualizes render durations commits, making it easier to spot components that are rendering too frequently or taking too long.
Native Profilers in Detail:
iOS: Xcode Instruments' Time Profiler helps identify CPU usage bottlenecks. Allocations helps spot memory leaks churn. Core Animation can visualize rendering performance frame rates.
Android: Android Studio Profiler offers insights into CPU, memory, network activity. systrace helps diagnose UI jank providing a detailed view of thread activity system calls over time.
Using a combination of JS native profiling tools offers a comprehensive view. Often, the root cause appears in one layer (e.g., JS) but manifests symptomatically in another (e.g., UI thread dropping frames).
Hermes Engine The Performance Boon
The shift to Hermes as the default JavaScript engine has been a salient advancement for React Native performance. Its AOT (Ahead-of-Time) compilation means less work is needed at runtime, leading to faster startup times lower memory footprint compared to engines like JSC (JavaScriptCore). Ensuring your project uses Hermes is step one in leveraging this performance advantage.
Optimizing App Size Startup Time
Minimizing the final APK/IPA size isn't just about disk space; it directly impacts download times, installation speed, crucially, startup time. The size of the JavaScript bundle, native libraries, assets all contribute. Techniques include:
Bundle Size Analysis: Tools like `react-native-bundle-visualizer` can parse Metro's bundle output show which modules contribute most to the bundle size, highlighting areas for optimization or potential code splitting.
Resource Minimization: Strip unused code (ProGuard R8 for Android, though RN handles much of this), minimize resource sizes (images, fonts), explore tools like App Thinning (iOS) Android App Bundles for delivering only necessary resources devices.
Expert Insights Forward-Looking Trends
The ecosystem is constantly evolving. Staying abreast of new architectural changes library updates is crucial for maintaining optimal cross-platform performance. "Performance optimization in React Native is a journey, not a destination. The architecture provides powerful primitives, but developer vigilance around bridging, rendering, and resource management remains key," notes an engineering lead I spoke with recently. This sentiment underscores the perpetual nature of performance work. Future developments, such as the ongoing rollout of Fabric (the new rendering system) TurboModules (the next generation of native modules), promise to significantly ameliorate performance bottlenecks associated with the traditional bridge by reducing the need for asynchronous serialization bridging overhead. Understanding these shifts and preparing your applications for their adoption is forward-thinking React Native optimization. While widespread production usage is still ramping up as of early 2025, monitoring their stability features is paramount.
Key Takeaways for Building High-Performing Apps
Creating performant React Native applications requires a disciplined and informed approach.
Prioritize Performance from the Outset: Consider performance implications early in design and development cycles.
Profile Meticulously: Regularly use profiling tools to identify actual bottlenecks, avoiding premature or misguided optimizations.
Optimize Component Rendering: Master `React.memo`, `useMemo`, `useCallback`, utilizing Virtualized Lists for large datasets.
Manage State Judiciously: Select state management patterns that minimize unnecessary re-renders updates.
Handle Data Efficiently: Implement caching, background processing for data fetching, optimize image handling.
Use Native Prudently Asynchronously: Move heavy computations to native code but ensure calls are non-blocking.
Monitor App Size Employ Hermes: Keep the bundle size minimal use the Hermes engine for faster startup better memory usage.
Frequently Asked Questions
What steps improve my React Native application lists speed? Strategies to boost rendering speed list views efficiently. Using virtualized list components such as FlatList SectionList is essential. Proper use of key extractorgetItemLayout helps significantly. How do I effectively manage vast data within my apps? Effective techniques for managing vast data in apps smoothly. Data virtualization via components like FlatList data pagination are primary methods. Cache data reduce network calls as well. Will utilizing the Hermes engine universally enhance performance? Understanding when utilizing Hermes engine improves app speed. Yes, for most modern applications, Hermes offers improved startup time and lower memory usage compared to other JS engines. Should native modules always be chosen for better performance? Determining suitability of integrating modules for performance boosts. No, native modules are suitable for CPU-bound tasks the JavaScript cannot handle efficiently, used prudently via asynchronous methods. What prevents the JavaScript thread from becoming blocked by operations? Keeping the core JS thread responsive and avoiding pauses. Avoid performing long-running or computationally heavy synchronous tasks on the JS thread. Use background processes web workers or asynchronous native modules instead.
Recommendations for Sustainable Performance
Building a fast React Native application is not a one-time task; it demands continuous attention. Integrate performance reviews into your development sprints. Treat performance metrics as seriously as functional requirements. Educate your development team on best practices and the nuances of the React Native architecture. Proactive monitoring deployment of efficient strategies outlined here will not only enhance user satisfaction but also reduce technical debt future refactoring costs. Make performance a core tenet of your React Native development philosophy starting today.
コメント